Supercharge Your Laravel Site Optimize Performance for Maximum Speed
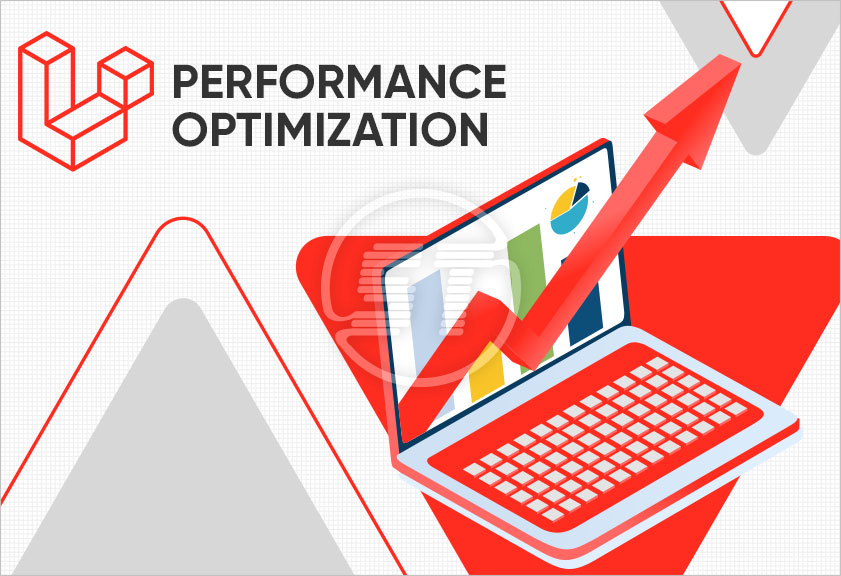
Laravel is one of the most popular PHP frameworks, providing developers with an expressive and elegant toolkit for building robust web applications, including those focused on Laravel website development. However, as Laravel applications grow in complexity, performance can suffer, leading to slow page loads and poor user experience.
By optimizing various aspects of your Laravel application, you”, “completion”:” achieve blazing fast performance. This article explores multiple techniques for squeezing every ounce of speed out of your Laravel site.
Overview: Performance Optimization for Laravel
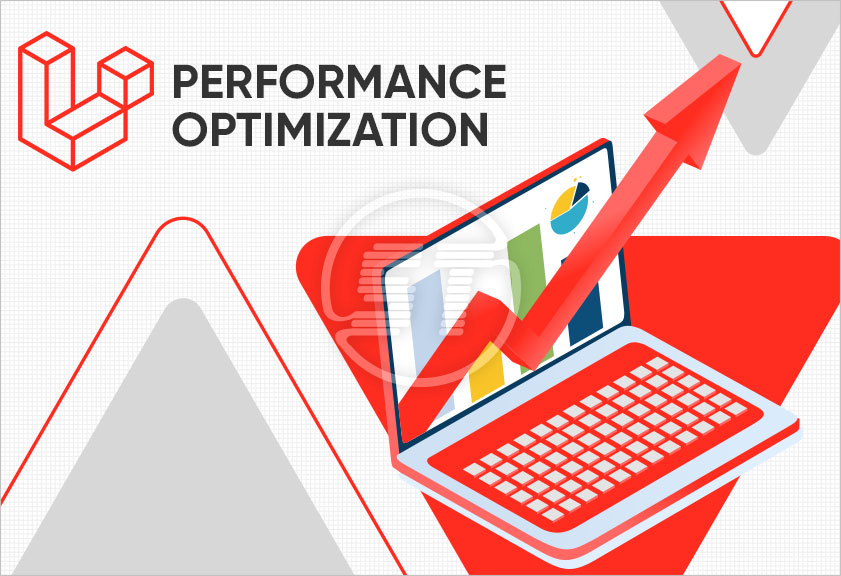
Performance optimization involves identifying and eliminating bottlenecks that hinder your application’s speed. For Laravel sites, common areas to optimize include:
- Database query performance
- Caching utilization
- Efficient routing
- Fine-tuned configuration
- Template rendering
- Event handling
- Error handling
- Deployment strategies
- Performance monitoring
When optimized holistically across these key areas, Laravel applications can deliver lightning-fast responses, even at high traffic volumes. Let’s explore each area in detail or visit https://alvacommerce.com/ for further exploration of Laravel development.
Optimizing Database Queries for Enhanced Performance
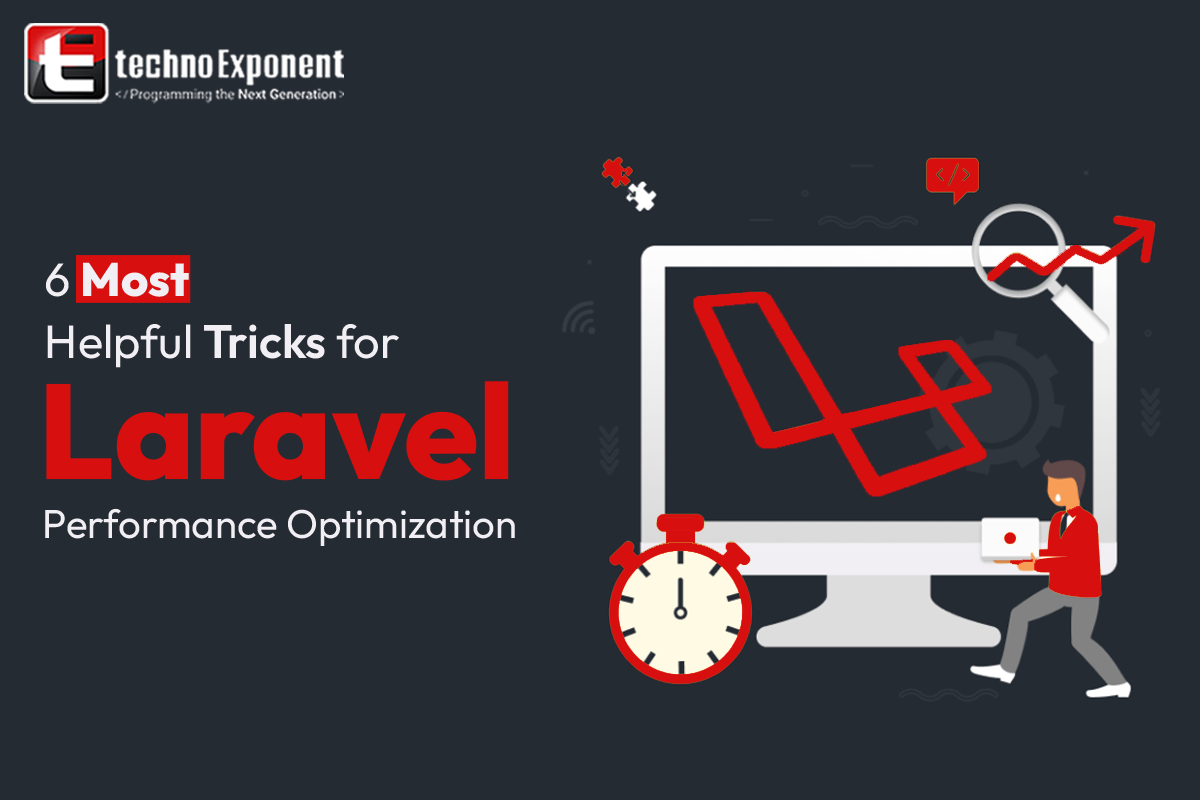
As a data-driven framework, database interactions play a pivotal role in Laravel application performance. Optimized database queries can retrieve data faster and reduce server load.
Using Eloquent Best Practices
Laravel’s Eloquent ORM provides a beautiful way to interact with databases. Here are some tips for writing blazing-fast Eloquent queries:
Lazy Loading Relations
Eager loading of related models upfront causes unnecessary queries:
// Eager loading
$books = Book::with('author', 'publisher')->get();
// Lazy loading
$books = Book::get();
$author = $books->first()->author;
Conditional Eager Loading
Only eager load when needed:
// Conditionally eager load
$books = Book::whenRequested()->with('author')->get();
Constraining Eager Loads
Limit unnecessary data with limit()
and take()
:
// Only take 1 publisher
$books->with(['publisher' => function($q){
$q","completion":"take(1);
}])->get();
following additional Eloquent optimizations:
- Select specific columns with
select()
to avoid grabbing unnecessary data - Use eager load constraints to reduce result sets
- Enable query logging to identify slow queries
Database Query Optimization
In addition to Eloquent best practices, broader database query optimization techniques include:
- Database indexing for faster lookups
- Caching”, “completion”:” repeat queries -“, “completion”: “Schema optimization to simplify joins
- Query analyzers to identify slow queries
Combined, these database optimizations can accelerate data access significantly.
Caching Strategies for Improved Application Speed
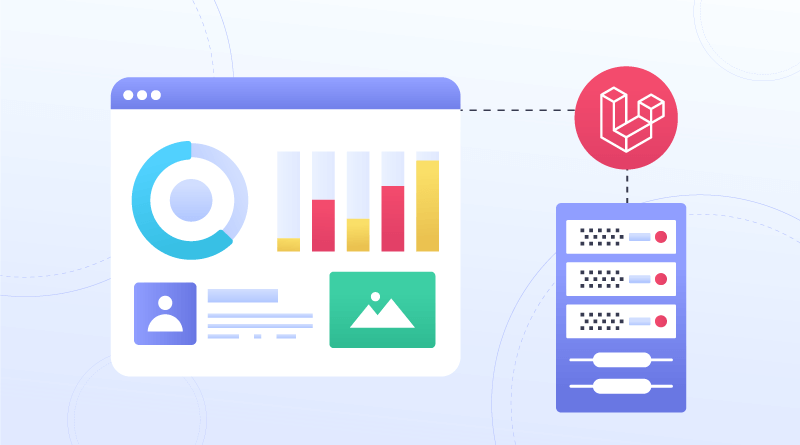
Caching avoids unnecessary database queries by storing frequently accessed data in memory. Laravel provides robust caching tools out of the box.
Cache Configuration Optimization
The cache configuration file governs caching behavior. Optimizations include:
- Driver selection – ApcU, Redis, or database
- Cache lifetime – Short for rapidly changing data
- Cache prefix – Avoid conflicts
- Multidriver – Different stores for different needs
For example:
// config/cache.php
'redis' => [
'driver' => 'redis',
'lifetime' => 3600,
'prefix' => 'laravel_',
],
Effective Cache Usage
To leverage caching effectively:
- Cache full pages
- Cache database queries
- Cache API requests
- Use cache tags for cache invalidation
- warmup cache after deployment
For example:
// Cache rendered view
return Cache::remember('home_page', 60, function(){
return view('home');
});
Common caching pitfalls include caching dynamic data incorrectly and not invalidating stale cache entries. Adopting an effective caching strategy suits most applications.
Essential Tips for Laravel Route Optimization
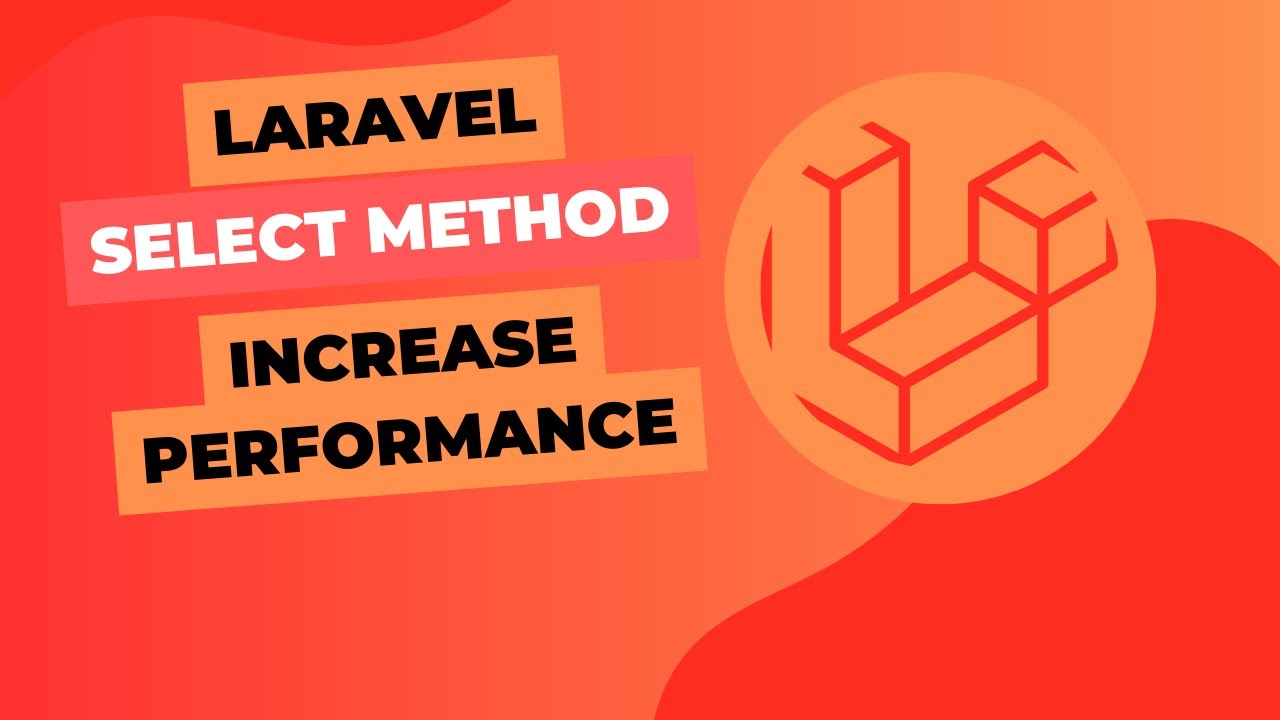
Routes dispatch incoming requests to controllers. Optimized routes allow requests to travel through the fastest possible path.
Route Configuration Best Practices
The routes/web.php file
governs routing configuration. Here are some optimizations:
Use Route Name Prefixes
Named routes with prefixes speed up lookup:
// Add admin prefix
Route::name('admin.')->prefix('admin')->group(function(){
Route::get('/users', 'UserController@index')->name('users');
});
Prefer Route Caching
All routes can be cached for faster registration:
// Boost speed with route caching
Route::get('/home', 'HomeController@index')->middleware('route.cache');
Route Ordering
Order routes from most specific to general for faster matching:
// Specific routes first
Route::get('/post/', 'PostController@show');
Route::get('/post/', 'PostController@search');
Two Routing Pitfalls to Avoid
Watch out for these common routing issues:
- Overusing route parameters leads to complex regex matching
- Defining too many catch-all routes
Following Laravel routing best practices avoids these pitfalls.
Fine-Tuning Laravel Configuration for Maximum Efficiency
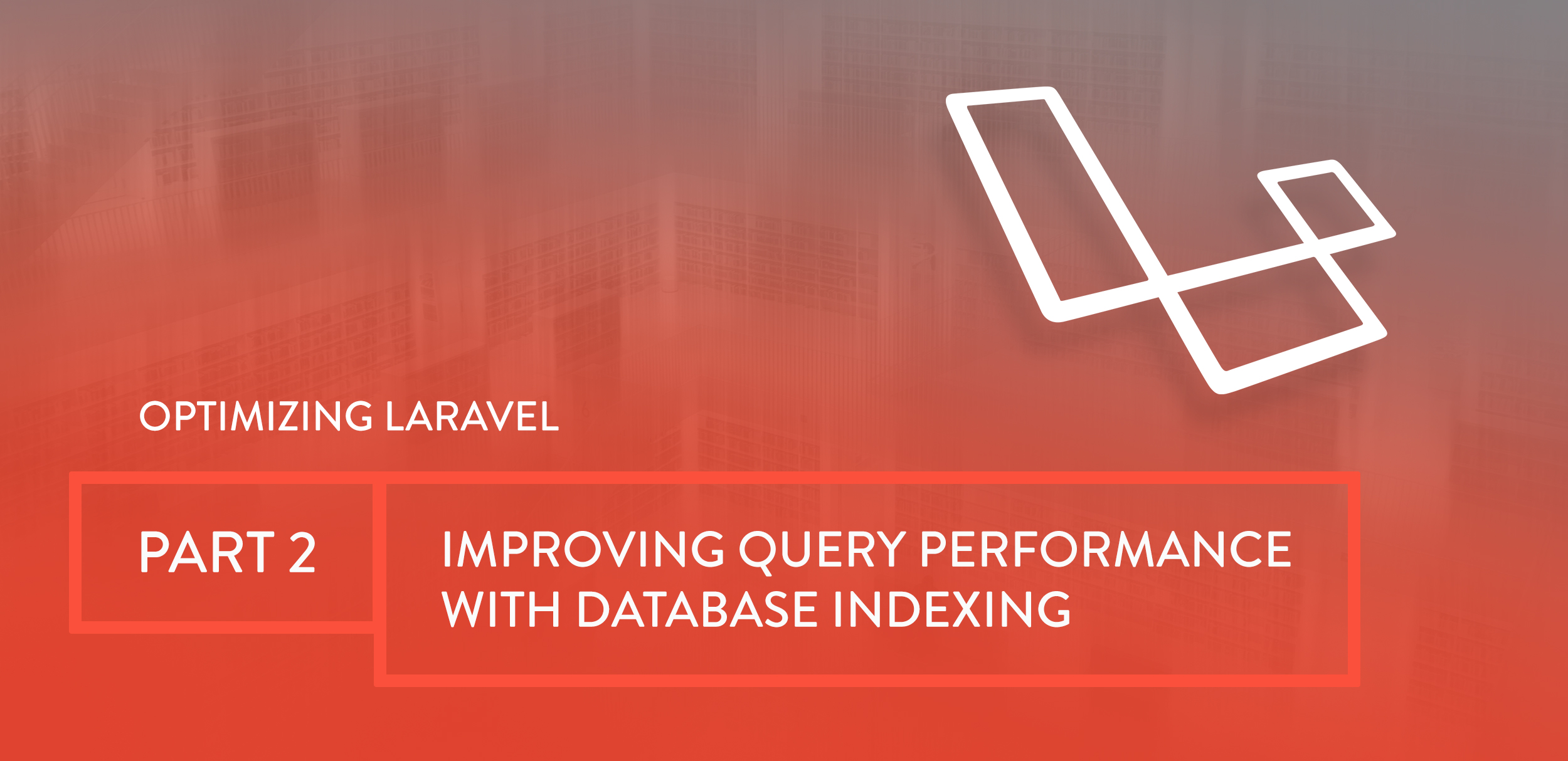
Laravel’s default configuration provides an excellent starting point for most applications. However, further tuning configuration to suit specific application characteristics can unlock extra performance gains.
Configuration Tuning Areas
Primary areas to fine-tune configuration include:
Cache Settings
As noted earlier, optimized cache configuration improves caching efficiency.
Logging Configuration
While crucial for debugging apps, excessive logging bogs down performance. Adjust log verbosity accordingly.
Queue Workers
If using queues for asynchronous tasks, tune worker numbers and connections appropriately.
Broadcasting
Real-time apps with WebSockets/broadcasting require capacity tuning.
Configuration Management
To ease configuration tuning, consider using the dotenv helper to manage environment-specific configuration, or even the Config helper to access configuration values dynamically.
Combined, adjusting these configuration areas can take your Laravel application speed to new heights!
Leveraging Blade Templates for Faster Rendering
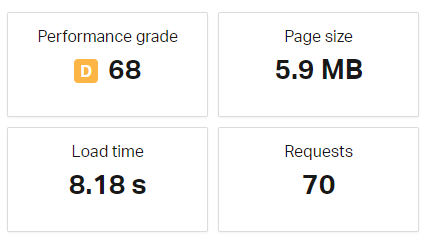
Laravel Blade provides a clean way of creating application UI using layouts, templates, components and slots. Well-architected Blade templates accelerate view rendering.
Tips for Faster Blade Rendering
Here”,”completion”:” some areas to focus on for faster Blade performance:
Template Inheritance
Nested template inheritance leads to slower rendering.Aim for shallow hierarchies.
Lean Templates
Keep templates minimal by abstracting shared UI into reusable components
Avoid Excess DOM Manipulation
Hand off DOM updates to JavaScript instead for snappy loading
Template Compilation
Precompile Blade templates to plain PHP to skip runtime compilation.
Identifying Slow Templates
Profile Blade performance by inspecting rendering times:
// See rendering times
dd(Profiler::get('views'));
Then optimize identified slow templates using the tips above. Simple yet performant Blade templates accelerate response times.
Event Optimization for Improved Laravel Performance
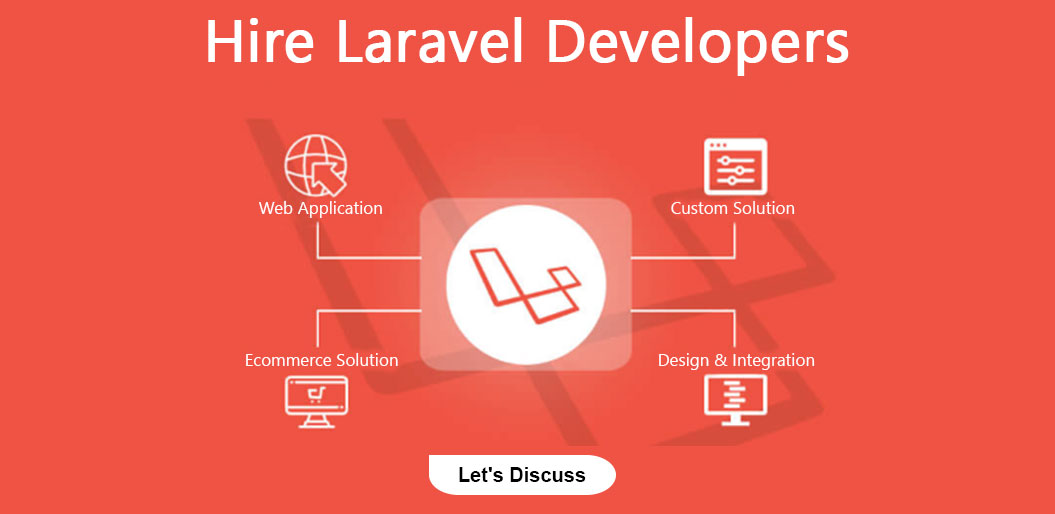
While events enable loose coupling, careless event usage hampers performance. Here are some tips for event optimization:
Using Events Judiciously
Only trigger events essential for the application flow. Avoid frequently firing events unexpectedly.
For example,”,”completion”:” events on every”,”completion”:” update overloads the system.
Checking for Listeners
Only fire events if active listeners exist:
// Avoid firing unless handled
if(Event::hasListeners('post.updated')) {
event(new PostUpdated($post));
}
Queueing Event Handlers
CPU-intensive event handlers should be queued:
// Queue this event handler
protected $listen = ['post.updated' => [
SendPostNotification::class . '@handle',
]];
This frees up the request handling thread.
Through mindful event usage, applications can react to system events without incurring performance penalties.
Effective Error Handling Techniques”,”completion”:” vital for diagnosing issues, poorly handled errors and exceptions degrade Laravel performance. Using appropriate error handling approaches solves this.
Controlled Exception Handling
Wrap risky code in try-catch blocks for controlled error flow:
try {
$user = User::findOrFail($id);
} catch (ModelNotFoundException $e) {
return error_response();
}
This avoids cascading failures.
Custom Exception Handling
Custom exception handling allows appropriate intervention:
public function render($request, Exception $exception){
if($exception instanceof CustomException){
// Handle custom exception
}
return parent::render(...);
}
Configured Logging
Log exceptions based on configured policies to avoid log spamming:
// Log only API errors
{
","completion":"channels": ["single", "slack"],
"only_exceptions": ["App\Exceptions\APIException"]
}
Robust error handling is crucial without hampering performance!
Deployment Best Practices for Enhanced Site Speed
Optimized deployment workflows take Laravel”,”completion”:” to the next level by eliminating friction during releases. Let’s explore some top deployment best practices.
Automated Build Pipelines
Automate builds by integrating tools like GitHub Actions/Circle CI into the code deploy process. This bakes in speed through consistency and reliability.
Example build workflow:
- Run tests
- Generate assets
- Deploy to servers
- Clear caches
Zero-Downtime Deployment
Use Blue-Green or Canary releasing rather than direct overwrite deployments. This eliminates downtime during new releases.
CDN Asset Caching
Serving assets through a blazing fast CDN accelerates asset load times especially for global users. Cloudflare and CloudFront are great CDN options.
Connection Pooling
Database connection pooling maintains persistent connections avoiding reconnects during deploy:
// .env file
DB_CONNECTION=mysql
DB_POOL=10
Embracing these and other best practices makes deployment faster and frustration-free.
Performance Monitoring and Profiling for Continuous Improvement
The hallmark of optimized Laravel applications is continuously monitoring performance and identifying areas for further enhancements.
Performance Metrics to Track
Some key application performance indicators to monitor include:
Metric | Tool |
---|---|
Page load times | Lighthouse |
Query counts | Laravel Debugbar |
| Error rates| Sentry |
Profiling with Debug Tools
Profile using debug tools during development”,”completion”:”
- Laravel Debugbar and Telescope for code profiling
- Blackfire.io for dedicated performance testing
Load Testing
Test real world load using tools like k6, Artillery.io and PHPUnit:
// PHPUnit test
public function testApplicationMax","completion":"()
{
// Load test logic
}
Together, purposeful monitoring and profiling ensures your Laravel app stays lean and blazing fast even as it evolves!
Video
Conclusion
While Laravel provides immense development velocity, application performance needs dedicated attention especially for large-scale applications. Fortunately, Laravel itself is designed for speed and provides all the right tools – from caching to queues – to turbocharge performance.
By holistically optimizing database queries, leveraging caching, improving templates efficiency, streamlining configuration and more, staggering performance gains can be realized making Laravel a true speed demon!
Deliberate performance monitoring provides the feedback needed to drive further enhancements. Both developers and users alike will appreciate the results – an incredibly snappy application able to handle enormous traffic volumes while delivering instantaneous responses!
So don’t settle for sluggish site performance – take action today to maximize speed with these Laravel performance best practices. Your users will thank you!